Feature Scaffolding
Code Snippets
Our VS Extension & VSCode Extension adds handy code snippet shortcuts to make it faster to scaffold/expand new classes in your project. JetBrains Rider users can import the snippets as LiveTemplates.
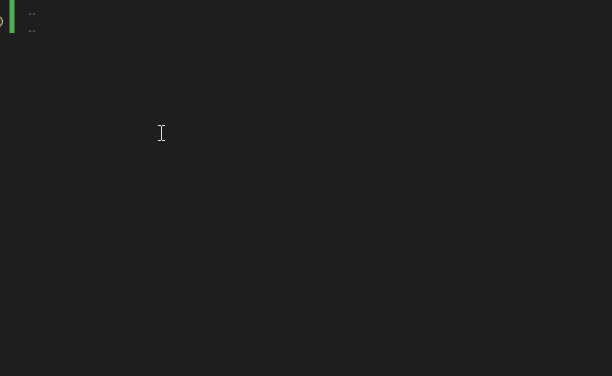
Available Shortcuts:
epreq
// Scaffolds an endpoint with only a request dto
sealed class Endpoint : Endpoint<Request>
epreqres
// Scaffolds an endpoint with request and response dtos
sealed class Endpoint : Endpoint<Request, Response>
epnoreq
// Scaffolds an endpoint without a request nor response dto
sealed class Endpoint : EndpointWithoutRequest
epres
// Scaffolds an endpoint without a request dto but with a response dto
sealed class Endpoint : EndpointWithoutRequest<Response>
epdto
// Scaffolds the request & response dtos for an endpoint
sealed class Request {}
sealed class Response {}
epval
// Scaffolds an endpoint validator for a given request dto
sealed class Validator : Validator<Request>
epmap
// Scaffolds an endpoint mapper class for the given request, response and entity dtos
sealed class Mapper : Mapper<Request, Response, Entity>
epsum
// Scaffolds a summary class for a given endpoint and request dto
sealed class Summary : Summary<Endpoint, Request>
epdat
// Scaffolds a static data class for an endpoint
static class Data
epfull
Scaffolds the complete set of classes for a full vertical slice
cmd
// Scaffolds a command handler for a given command model that does not return a result
sealed class CommandHandler : ICommandHandler<Command>
cmdres
// Scaffolds a command handler for a given command model that returns a result
sealed class CommandHandler : ICommandHandler<Command, Result>
evnt
// Scaffolds an event handler for a given event model
sealed class EventHandler : IEventHandler<Event>
preproc
// Scaffolds a pre-processor for a given request dto
sealed class Processor : IPreProcessor<Request>
postproc
// Scaffolds a post-processor for a given request & response dto
sealed class Processor : IPostProcessor<Request, Response>
Integration Test Scaffolds
- tstfixture - scaffolds a test class fixture
- tstclass - scaffolds a test class
- tstmethod - scaffolds a test method with a [Fact] attribute
VS New Item Template
If you're doing vertical slice architecture and placing each individual feature in their own namespace, you can take advantage of the VS Extension that will add a new item to the "add new file" dialog of visual studio to make it convenient to add feature file sets to your project.
Once installed, your visual studio add new item dialog will have FastEndpoints Feature File Set listed under Installed > Visual C# node. Then, instead of entering a file name, simply enter the namespace you want your new feature to be added to followed by .cs
A new feature file set will be created in the folder you selected.
There will be 4 new files created under the namespace you chose.
Data.cs - Use this class to place all of your data access logic.
Models.cs - Place your request, response DTOs and the validator in this file.
Mapper.cs - Domain entity mapping logic will live here.
Endpoint.cs - This will be your new endpoint definition.
Click here for an example feature file set.
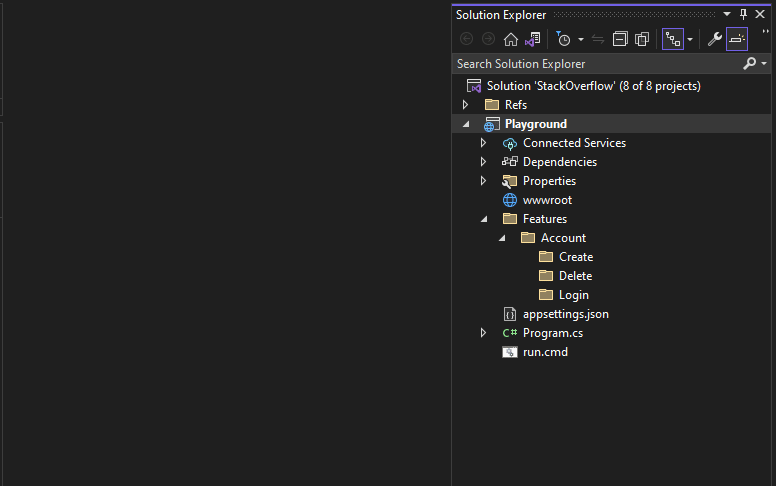
Dotnet New Item Template
If you prefer working with the cli, you can use our dotnet new template to create a new feature file set.
dotnet new install FastEndpoints.TemplatePack
Usage:
The example feature below will use the following input parameters:
- Namespace: MyProject.Comments.Create
- Method POST
- Route: api/comments
Files will be created in folder Features/Comments/Create:
dotnet new feat -n MyProject.Comments.Create -m post -r api/comments -o Features/Comments/Create
Available Options
> dotnet new feat --help
FastEndpoints Feature Fileset (C#)
Options:
-t|--attributes Whether to use attributes for endpoint configuration
bool - Optional
Default: false
-p|--mapper Whether to use a mapper
bool - Optional
Default: true
-v|--validator Whether to use a validator
bool - Optional
Default: true
-m|--method Endpoint HTTP method
GET
POST
PUT
DELETE
PATCH
Default: GET
-r|--route Endpoint path
string - Optional
Default: api/route/here
Project Scaffolding
In order to scaffold new projects, install the Template Pack if not already installed:
dotnet new install FastEndpoints.TemplatePack
Bare-Bones Starter Project Template
A new FastEndpoints starter project including traditional integration testing setup with xUnit:
dotnet new feproj -n MyAwesomeProject
Integrated Testing Project Template
An alternate starter project that places xUnit tests alongside the endpoints that are being tested:
dotnet new feintproj -n MyAwesomeProject
xUnit Test Project
A xUnit integration testing project:
dotnet new fetest
VS New Project Dialog
After the template pack is installed with dotnet new, the project templates will show up in Visual Studio as well.
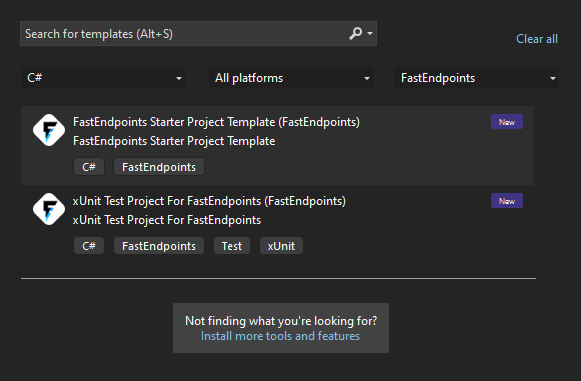
OpenAPI Generator
OpenAPI Generator is a CLI tool that can generate a FastEndpoints server project from a given OpenAPI document. To install the tool, please follow the instructions on the Getting Started page. Once installed, a project can be generated using an OpenAPI document as follows:
openapi-generator-cli generate \
--generator-name aspnet-fastendpoints \
--input-spec d:/my_open_api_file.yaml \
--output d:/my_fastendpoints_project
A complete list of parameters can be found in the generator's documentation. This is a community contribution. please visit the author's github repository for feature requests and bug reports.